Introduction
This is the chapter web page to support the content in Chapter 12 of the book: Exploring Raspberry Pi – Interfacing to the Real World with Embedded Linux. The summary introduction to the chapter is as follows:
This chapter describes how the Raspberry Pi can be used as a core building block of the Internet of Things (IoT). In this chapter, you are introduced to the concepts of network programming, the IoT, and the connection of sensors to the Internet. Several different communications architectures are described: The first architecture configures the RPi to be a web server that uses various server-side scripting techniques to display sensor data. Next, custom C/C++ code is described that can push sensor data to the Internet and to platform as a service (PaaS) offerings, such as ThingSpeak and the IBM Bluemix IoT service (using MQTT). Finally, a client/server pair for high-speed Transmission Control Protocol (TCP) socket communication is described. The latter part of the chapter introduces some techniques for managing distributed RPi sensors, and physical networking topics: setting the RPi to have a static IP address; and using Power over Ethernet (PoE) with the RPi. By the end of this chapter, you should be able to build your own full-stack IoT devices.
After completing this chapter, you should hopefully be able to do the following:
- Install and configure a web server on the RPi and use it to display static HTML content.
- Enhance the web server to send dynamic web content that uses CGI scripts and PHP scripts to interface to RPi sensors.
- Write the code for a C/C++ client application that can communicate using either HTTP or HTTPS.
- Interface to platform as a service (PaaS) offerings, such as ThingSpeak and IBM Bluemix IoT, using HTTP and MQTT.
- Use the Linux cron scheduler to structure workflow on the RPi.
- Send e-mail messages directly from the RPi and utilize them as a trigger for web services such as IFTTT.
- Build a C++ client/server application that can communicate at a high speed and a low overhead between any two TCP devices.
- Manage remote RPi devices, using monitoring software and watchdog code, to ensure that deployed services are robust.
- Configure the RPi to use Wi-Fi adapters and static IP addresses, and wire the RPi to utilize Power over Ethernet (PoE).
Updates
Updates to the Paho Project
The paho project that is described on page 514/515 has moved from git.eclipse.org to github.com. You can follow the steps in the chapter by changing the git URI as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
molloyd@molloyd-VirtualBox:~/$ git clone https://github.com/eclipse/paho.mqtt.c Cloning into 'paho.mqtt.c'... remote: Counting objects: 2220, done. remote: Total 2220 (delta 0), reused 0 (delta 0), pack-reused 2220 Receiving objects: 100% (2220/2220), 875.23 KiB | 1.17 MiB/s, done. Resolving deltas: 100% (1573/1573), done. Checking connectivity... done. molloyd@molloyd-VirtualBox:~/$ cd paho.mqtt.c/ molloyd@molloyd-VirtualBox:~/paho.mqtt.c$ make mkdir -p build/output/samples mkdir -p build/output/test echo OSTYPE is Linux OSTYPE is Linux ... molloyd@molloyd-VirtualBox:~/paho.mqtt.c$ sudo make install |
Digital Media Resources
Below are some high-resolution images of the circuits described in the book. They are reproduced in colour and can be printed at high resolution to facilitate you in building the circuits.
The Client/Server Models Described in the Chapter
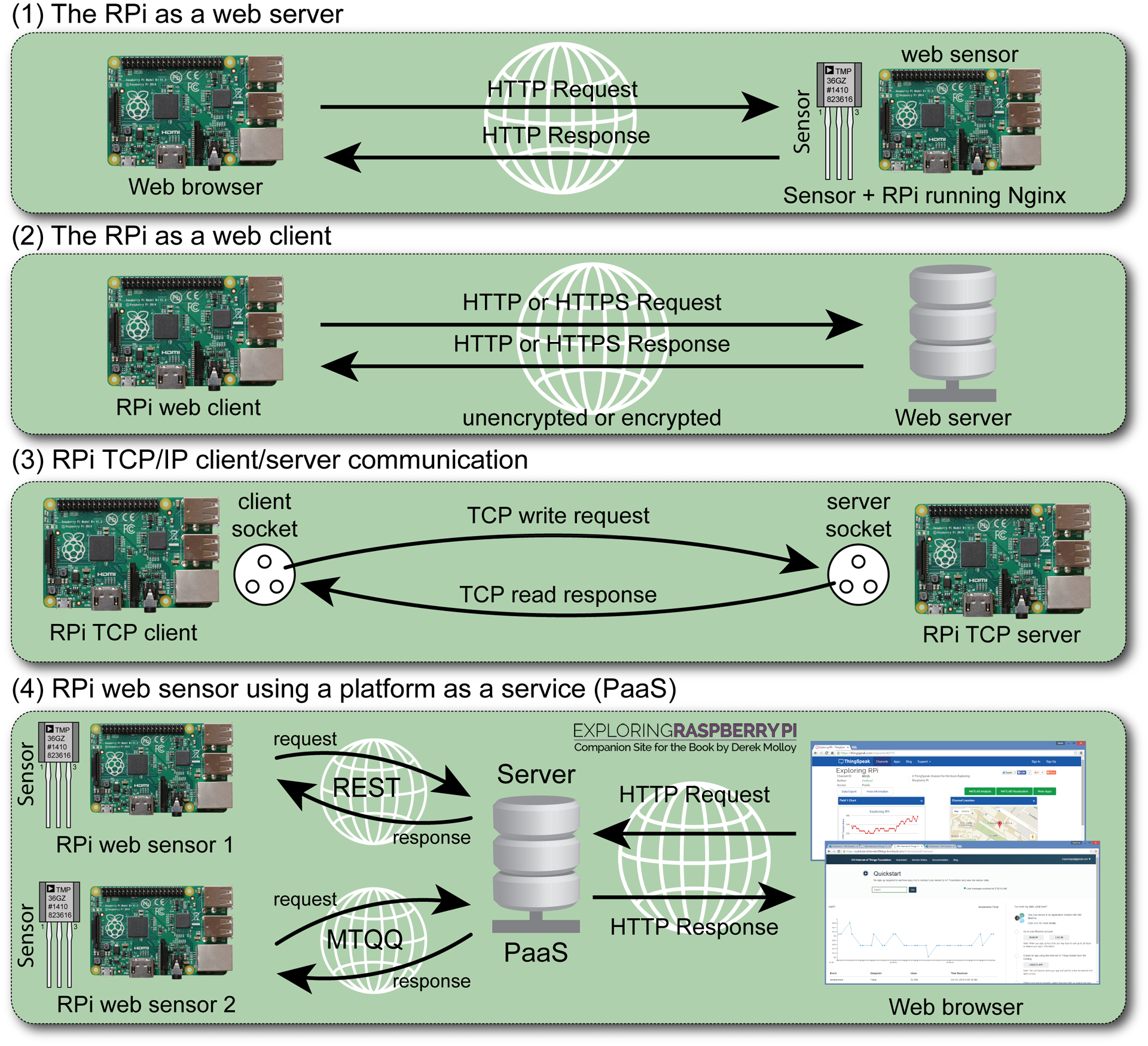
Heatsinks and the RPi
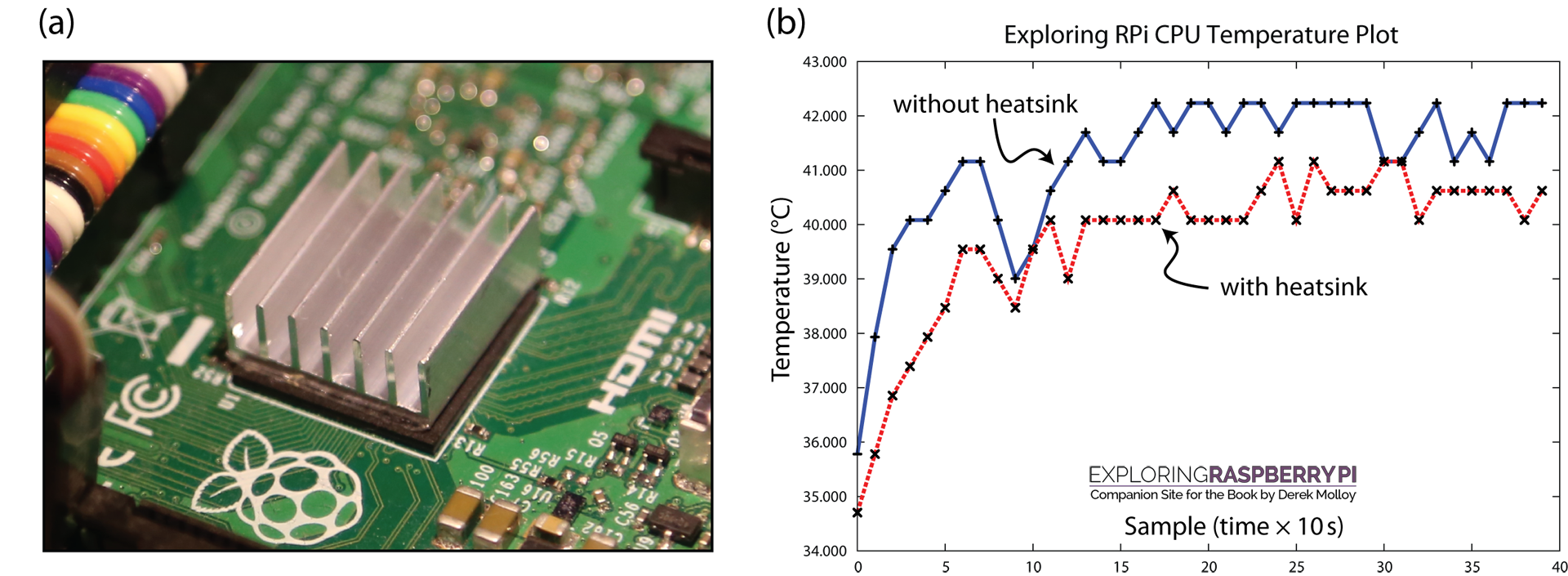
The IBM Watson Bluemix IoT Example
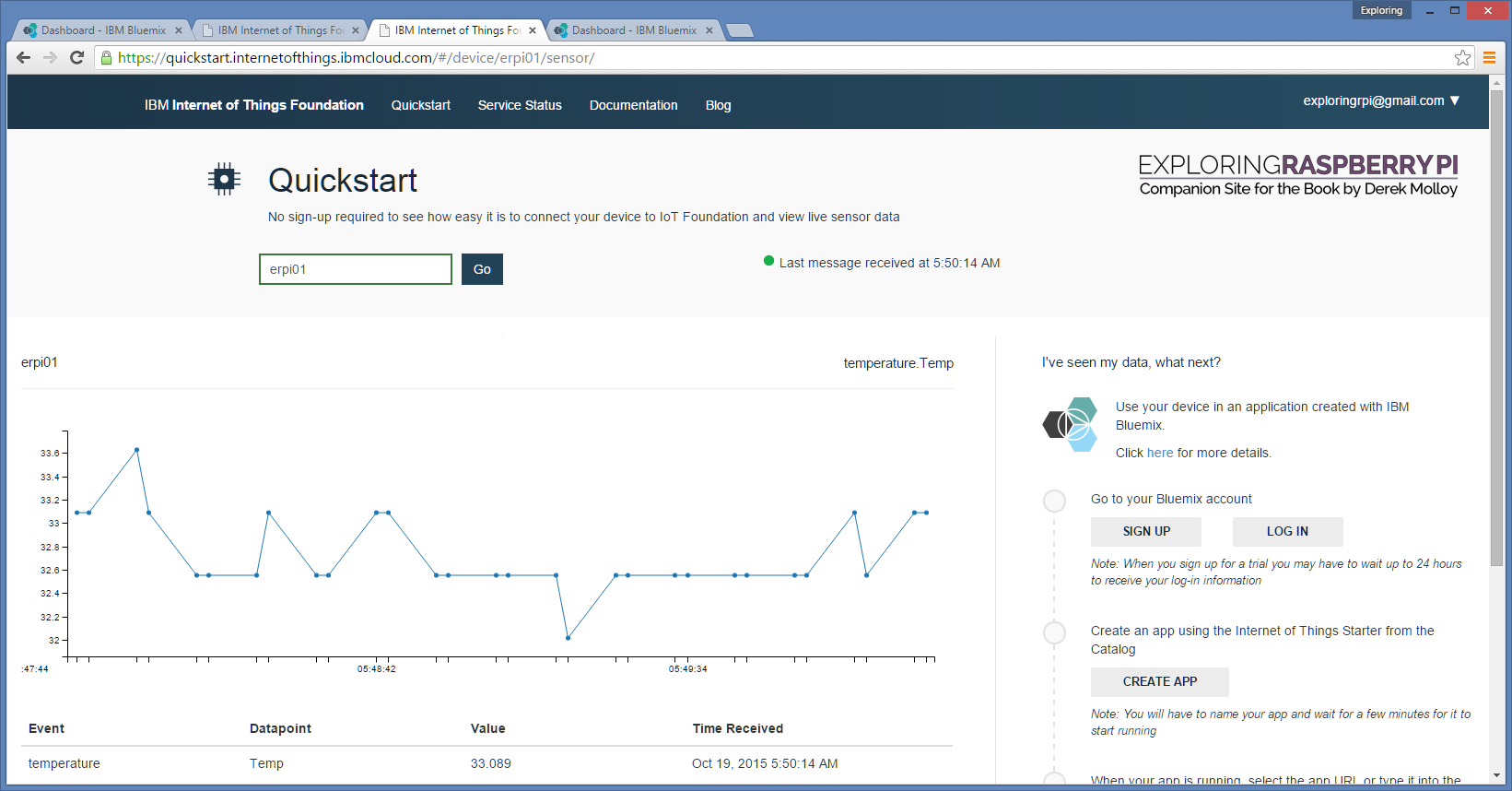
Errata
None for the moment
Recommended Books on the Content in this Chapter
In Listing 12.6 I believe the line
SocketClient sc( “thingpeak.com”,80);
should be
SocketClient sc(“api.thingspeak.com”, 80);
Thanks, it must have been updated and that is very useful to know. Kind regards, Derek.
Hi Derek,
Love your book. Love it to bits. But I got stuck on page 502 trying to install OpenSSL.
pi@raspberrypi:~ $ sudo apt install openssl libssl-dev
Reading package lists… Done
Building dependency tree
Reading state information… Done
openssl is already the newest version.
The following NEW packages will be installed:
libssl-dev libssl-doc
0 upgraded, 2 newly installed, 0 to remove and 0 not upgraded.
Need to get 0 B/2,262 kB of archives.
After this operation, 6,480 kB of additional disk space will be used.
Selecting previously unselected package libssl-dev:armhf.
dpkg: unrecoverable fatal error, aborting:
unable to open files list file for package `libvorbisfile3:armhf’: Structure needs cleaning
E: Sub-process /usr/bin/dpkg returned an error code (2)
Steve
RPi3, default O/S, Linux newbie
Hello derek,
On your example for “IBM IoT MQTT C++” on page 520, the code exits with an rc state of 5.
I cannot figure out the issue with the same…
#include
#include
#include
#include
#include "MQTTClient.h"
#define CPU_TEMP "/sys/class/thermal/thermal_zone0/temp"
using namespace std;
#define ADDRESS "tcp://(MY_URL).messaging.internetofthings.ibmcloud.com:1883"
#define CLIENTID "d:(MY_URL):RaspberryPi:erpi01"
#define AUTHMETHOD "use-token-auth"
#define AUTHTOKEN "(MY_TOKEN)"
#define TOPIC "iot-2/evt/status/fmt/json"
#define QOS 1
#define TIMEOUT 10000L
float getCPUTemperature(){
int CPUTemp;
fstream fs;
fs.open(CPU_TEMP, fstream::in);
fs >> CPUTemp;
fs.close();
return (((float)CPUTemp)/1000);
}
int main(int argc, char* argv[]) {
MQTTClient client;
MQTTClient_connectOptions opts = MQTTClient_connectOptions_initializer;
MQTTClient_message pubmsg = MQTTClient_message_initializer;
MQTTClient_deliveryToken token;
MQTTClient_create(&client, ADDRESS, CLIENTID, MQTTCLIENT_PERSISTENCE_NONE, NULL);
opts.keepAliveInterval = 20;
opts.cleansession = 1;
opts.username = AUTHMETHOD;
opts.password = AUTHTOKEN;
int rc;
if ((rc = MQTTClient_connect(client, &opts)) != MQTTCLIENT_SUCCESS)
{
cout<<"Failed to connect "<<rc<<endl;
return -1;
}
stringstream message;
message <<"{\"d\":{\"Temp\":"<<getCPUTemperature()<<"}}";
pubmsg.payload = (char*) message.str().c_str();
pubmsg.payloadlen = message.str().length();
pubmsg.qos = QOS;
pubmsg.retained = 0;
MQTTClient_publishMessage(client, TOPIC, &pubmsg, &token);
cout<< "Waiting for " << (int) (TIMEOUT/1000) << "seconds for pub of"<<message.str()<<"\non topic"<<TOPIC<<"for ClientID:"<<CLIENTID<<endl;
rc = MQTTClient_waitForCompletion(client, token, TIMEOUT);
cout<<"Message with token"<< (int)token<<"delivered"<<endl;
MQTTClient_disconnect(client,10000);
MQTTClient_destroy(&client);
return rc;
}
Do we have to do anything else after creating a device on IBM bluemix..??
Frankly they have changed the entire interface of bluemix.
On page 510, the setting “hostname=myaccountname@gmail.com” in /etc/ssmtp/ssmtp.config caused the error “ssmtp: Cannot open smtp.gmail.com:587”. Changing it to “hostname=raspberrypi” which is the hostname of my raspberry pi corrected the problem.
PHP5 does not install anymore, should update to php7.0 on commands listed on page 493.